Authentication
FlexFactor uses API keys to authenticate requests. Every subsequent API request must contain a bearer token in the header.
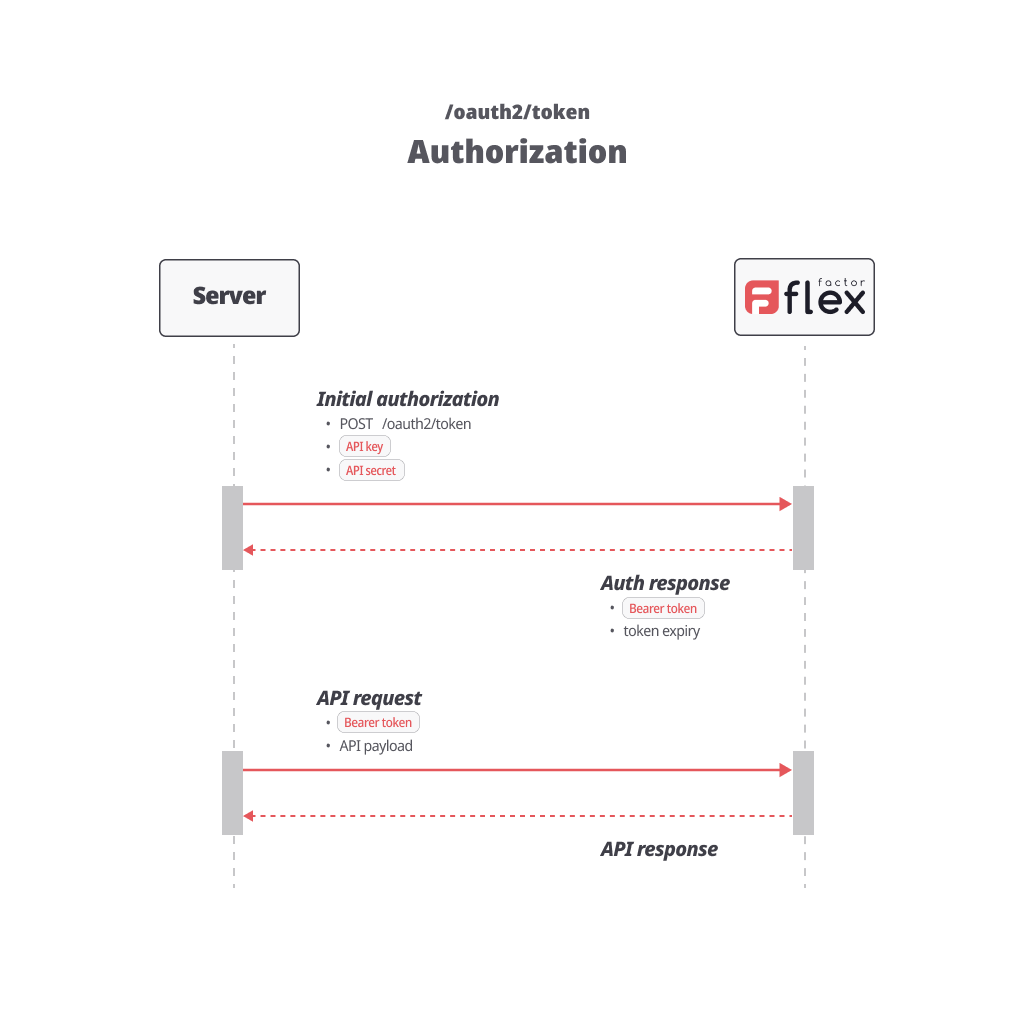
Request
curl --location -g --request POST '{URL}/oauth2/token' \
--header 'Content-Type: application/json' \
--data-raw '{
"AppKey": "{Replace with your AppKey found on the portal}",
"AppSecret": "{Replace with your AppSecret found on the portal}"
}'
var axios = require('axios');
var data = JSON.stringify({
"client_id": "d572283e-1cd2-4c8b-9944-6d994ffc120e",
"client_secret": "LLw_oYT_zDsV46Cq_wuOGw"
});
var config = {
method: 'post',
url: '{{URL}}/oauth2/token',
headers: {
'Content-Type': 'application/json'
},
data : data
};
axios(config)
.then(function (response) {
console.log(JSON.stringify(response.data));
})
.catch(function (error) {
console.log(error);
});
Endpoints
Sandbox testing | https://api-sandbox.flexfactor.io/v1/](tps://api-sandbox.flexfactor.io/v1/ |
Production environment | https://api.flexfactor.io/v1/](tps://api.flexfactor.io/v1/ |
Required keys
These keys will be used for retrieving a bearer token.
| The public Client ID used to identify your App. |
| The Client Secret used to authenticate. |
Log into into your Sandbox. Go to section Developers > Api Keys get both your AppKey
and AppSecret
.
Response
| JSON Web Token (JWT) that contains information your |
| A longer-lived token that's used to obtain a new access token without needing to re-enter credentials. You can use this token to request a new access token once the current access token expires. |
| Timestamp indicating when the |
Token expires after 10 minutes from generation time.Do not request a new token for each API request. Please use the current token until you receive a 401 unauthorized error, at which point you can request a new token.
Response example
{
"accessToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiJmZjVlOGNhYS04MTIyLTQyOTUtODVkZi1kOWRkYmNhMmU1NTMiLCJ1bmlxdWVfbmFtZSI6ImZmNWU4Y2FhLTgxMjItNDI5NSasdadsGRiY2EyZTU1MyIsImp0aSI6ImQwYzQzYjlkLWVhZDktNDFjNS1hMmFiLTBlZWVjNDQwNGUwMiIsImlhdCI6IjE2NjExNTcwNjg3OTIiLCJhdWQiOiJlbGlnaWJpbGl0eS1zZXJ2aWNlIiwiY3VzdG9tOm1pZCI6IjY1Zjg2YmY1LTk1NDYtNGRjMS1hNGIyLWE5MWIzOWMxODNkNCIsIm5iZiI6MTY2MTE1NzA2OCwiZXhwIjoxNjYxMTU3NjY4LCJpc3MiOiJBcGktQ2xpZW50LVNlcnZpY2UifQ.sFuj_ia3u0kM15oVmvXMLJSL60-raqk1IMKtlGqciE4",
"refreshToken": "AQAAsadEAACcQAdsadUSgIrIRsFwgasdaspG2lVCBCO59WMUmYTG4qt3iFRBhNh4AHksd6Mw",
"expires": 1620157668792,
"id": "ff5e8caa-8122-4222-851f-d9dasca2e553",
"success": true,
"result": null,
"status": null,
"statusCode": null,
"errors": [],
"customProperties": {}
}
Updated 23 days ago